24.1.26. Vector selection
24.1.26.1. Extract by attribute
Creates two vector layers from an input layer: one will contain only matching features while the second will contain all the non-matching features.
The criteria for adding features to the resulting layer is based on the values of an attribute from the input layer.
See also
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[vector: any] |
Layer to extract features from. |
Selection attribute |
|
[tablefield: any] |
Filtering field of the layer |
Operator |
|
[enumeration] Default: 0 |
Many different operators are available:
|
Value Optional |
|
[string] |
Value to be evaluated |
Extracted (attribute) |
|
[same as input] Default: |
Specify the output vector layer for matching features. One of:
The file encoding can also be changed here. |
Extracted (non-matching) |
|
[same as input] Default: |
Specify the output vector layer for non-matching features. One of:
The file encoding can also be changed here. |
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Extracted (attribute) |
|
[same as input] |
Vector layer with matching features from the input layer |
Extracted (non-matching) |
|
[same as input] |
Vector layer with non-matching features from the input layer |
Python code
Algorithm ID: qgis:extractbyattribute
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.26.2. Extract by expression
Creates two vector layers from an input layer: one will contain only matching features while the second will contain all the non-matching features.
The criteria for adding features to the resulting layer is based on a QGIS expression. For more information about expressions see the Expressions.
See also
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[vector: any] |
Input vector layer |
Expression |
|
[expression] |
Expression to filter the vector layer |
Matching features |
|
[same as input] Default: |
Specify the output vector layer for matching features. One of:
The file encoding can also be changed here. |
Non-matching |
|
[same as input] Default: |
Specify the output vector layer for non-matching features. One of:
The file encoding can also be changed here. |
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Matching features |
|
[same as input] |
Vector layer with matching features from the input layer |
Non-matching |
|
[same as input] |
Vector layer with non-matching features from the input layer |
Python code
Algorithm ID: qgis:extractbyexpression
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.26.3. Extract by location
Creates a new vector layer that only contains matching features from an input layer.
The criteria for adding features to the resulting layer is based on the spatial relationship between each feature and the features in an additional layer.
See also
Exploring spatial relations
Geometric predicates are boolean functions used to determine the spatial relation a feature has with another by comparing whether and how their geometries share a portion of space.
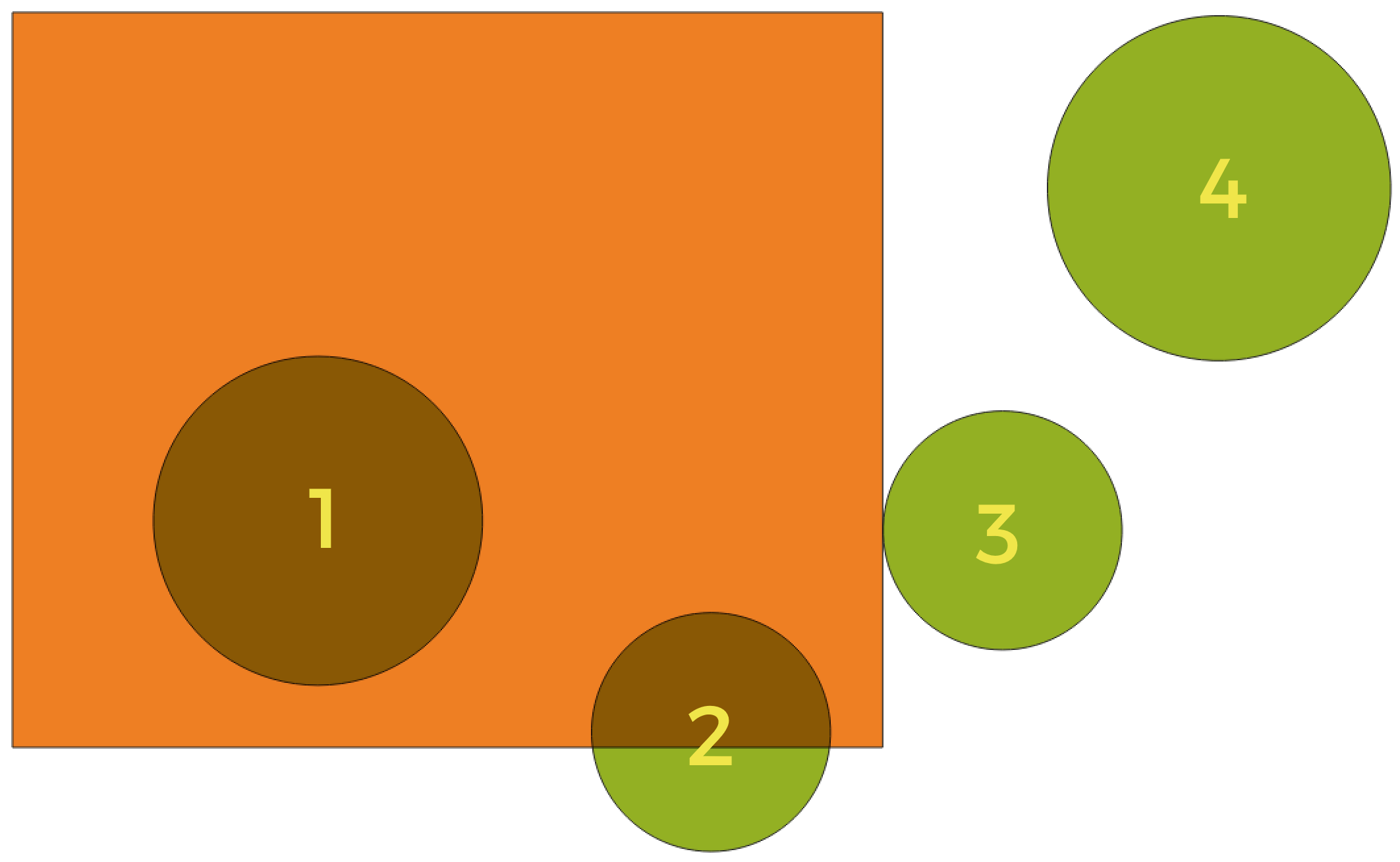
Fig. 24.133 Looking for spatial relations between layers
Using the figure above, we are looking for the green circles by spatially comparing them to the orange rectangle feature. Available geometric predicates are:
- Intersect
Tests whether a geometry intersects another. Returns 1 (true) if the geometries spatially intersect (share any portion of space - overlap or touch) and 0 if they don’t. In the picture above, this will return circles 1, 2 and 3.
- Contain
Returns 1 (true) if and only if no points of b lie in the exterior of a, and at least one point of the interior of b lies in the interior of a. In the picture, no circle is returned, but the rectangle would be if you would look for it the other way around, as it contains circle 1 completely. This is the opposite of are within.
- Disjoint
Returns 1 (true) if the geometries do not share any portion of space (no overlap, not touching). Only circle 4 is returned.
- Equal
Returns 1 (true) if and only if geometries are exactly the same. No circles will be returned.
- Touch
Tests whether a geometry touches another. Returns 1 (true) if the geometries have at least one point in common, but their interiors do not intersect. Only circle 3 is returned.
- Overlap
Tests whether a geometry overlaps another. Returns 1 (true) if the geometries share space, are of the same dimension, but are not completely contained by each other. Only circle 2 is returned.
- Are within
Tests whether a geometry is within another. Returns 1 (true) if geometry a is completely inside geometry b. Only circle 1 is returned.
- Cross
Returns 1 (true) if the supplied geometries have some, but not all, interior points in common and the actual crossing is of a lower dimension than the highest supplied geometry. For example, a line crossing a polygon will cross as a line (true). Two lines crossing will cross as a point (true). Two polygons cross as a polygon (false). In the picture, no circles will be returned.
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Extract features from |
|
[vector: geometry] |
Input vector layer |
Where the features (geometric predicate) |
|
[enumeration] [list] Default: [0] |
Type of spatial relation the input feature should have with an intersect feature so that it could be selected. One or more of:
If more than one condition is chosen, at least one of them (OR operation) has to be met for a feature to be extracted. |
By comparing to the features from |
|
[vector: geometry] |
Intersection vector layer |
Extracted (location) |
|
[same as input] Default: |
Specify the output vector layer for the features that have the chosen spatial relationship(s) with one or more features in the comparison layer. One of:
The file encoding can also be changed here. |
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Extracted (location) |
|
[same as input] |
Vector layer with features from the input layer that have the chosen spatial relationship(s) with one or more features in the comparison layer. |
Python code
Algorithm ID: qgis:extractbylocation
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.26.4. Extract within distance
Creates a new vector layer that only contains matching features from an input layer. Features are copied wherever they are within the specified maximum distance from the features in an additional reference layer.
See also
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Extract features from |
|
[vector: geometry] |
Input vector layer to copy features from |
By comparing to the features from |
|
[vector: geometry] |
Vector layer whose features closeness is used |
Where the features are within |
|
Default: 100.0 |
The maximum distance around reference features to select input features within |
Modify current selection by |
|
[enumeration] Default: 0 |
How the selection of the algorithm should be managed. One of:
|
Extracted (location) |
|
[same as input] Default: |
Specify the output vector layer for the features that are within the set distance from reference features. One of:
The file encoding can also be changed here. |
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Extracted (location) |
|
[same as input] |
Vector layer with features from the input layer matching the condition of distance from reference features |
Python code
Algorithm ID: native:extractwithindistance
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.26.5. Filter by geometry type
Filters features by their geometry type. Incoming features will be directed to different outputs based on whether they have a point, line or polygon geometry.
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[vector: any] |
Layer to evaluate |
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Point features Optional |
|
[vector: point] |
Layer with points |
Line features Optional |
|
[vector: line] |
Layer with lines |
Polygon features Optional |
|
[vector: polygon] |
Layer with polygons |
Features with no geometry Optional |
|
[vector: table] |
Geometry-less vector layer |
Python code
Algorithm ID: native:filterbygeometry
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.26.6. Random extract
Takes a vector layer and generates a new one that contains only a subset of the features in the input layer.
The subset is defined randomly, based on feature IDs, using a percentage or count value to define the total number of features in the subset.
Warning
This algorithm drops existing primary keys or FID values and regenerate them in output layers.
See also
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[vector: any] |
Source vector layer to select the features from |
Method |
|
[enumeration] Default: 0 |
Random selection methods. One of:
|
Number/percentage of selected features |
|
[numeric: integer] Default: 10 |
Number or percentage of features to select |
Extracted (random) |
|
[same as input] Default: |
Specify the output vector layer for the randomly selected features. One of:
The file encoding can also be changed here. |
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Extracted (random) |
|
[same as input] |
Vector layer containing randomly selected features from the input layer |
Python code
Algorithm ID: qgis:randomextract
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.26.7. Random extract within subsets
Takes a vector layer and generates a new one that contains only a subset of the features in the input layer.
The subset is defined randomly, based on feature IDs, using a percentage or count value to define the total number of features in the subset. The percentage/count value is not applied to the whole layer, but instead to each category. Categories are defined according to a given attribute.
See also
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[vector: any] |
Vector layer to select the features from |
ID field |
|
[tablefield: any] |
Category of the source vector layer to select the features from |
Method |
|
[enumeration] Default: 0 |
Random selection method. One of:
|
Number/percentage of selected features |
|
[numeric: integer] Default: 10 |
Number or percentage of features to select |
Extracted (random stratified) |
|
[same as input] Default: |
Specify the output vector layer for the randomly selected features. One of:
The file encoding can also be changed here. |
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Extracted (random stratified) |
|
[same as input] |
Vector layer containing randomly selected features from the input layer |
Python code
Algorithm ID: qgis:randomextractwithinsubsets
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.26.8. Random selection
Takes a vector layer and selects a subset of its features. No new layer is generated by this algorithm.
The subset is defined randomly, based on feature IDs, using a percentage or count value to define the total number of features in the subset.
Default menu:
See also
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[vector: any] |
Vector layer for the selection |
Method |
|
[enumeration] Default: 0 |
Random selection method. One of:
|
Number/percentage of selected features |
|
[numeric: integer] Default: 10 |
Number or percentage of features to select |
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[same as input] |
The input layer with features selected |
Python code
Algorithm ID: qgis:randomselection
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.26.9. Random selection within subsets
Takes a vector layer and selects a subset of its features. No new layer is generated by this algorithm.
The subset is defined randomly, based on feature IDs, using a percentage or count value to define the total number of features in the subset.
The percentage/count value is not applied to the whole layer, but instead to each category.
Categories are defined according to a given attribute, which is also specified as an input parameter for the algorithm.
No new outputs are created.
Default menu:
See also
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[vector: any] |
Vector layer to select features in |
ID field |
|
[tablefield: any] |
Category of the input layer to select the features from |
Method |
|
[enumeration] Default: 0 |
Random selection method. One of:
|
Number/percentage of selected features |
|
[numeric: integer] Default: 10 |
Number or percentage of features to select |
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[same as input] |
The input layer with features selected |
Python code
Algorithm ID: qgis:randomselectionwithinsubsets
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.26.10. Select by attribute
Creates a selection in a vector layer.
The criteria for selecting features is based on the values of an attribute from the input layer.
See also
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[vector: any] |
Vector layer to select features in |
Selection attribute |
|
[tablefield: any] |
Filtering field of the layer |
Operator |
|
[enumeration] Default: 0 |
Many different operators are available:
|
Value Optional |
|
[string] |
Value to be evaluated |
Modify current selection by |
|
[enumeration] Default: 0 |
How the selection of the algorithm should be managed. One of:
|
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[same as input] |
The input layer with features selected |
Python code
Algorithm ID: qgis:selectbyattribute
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.26.11. Select by expression
Creates a selection in a vector layer.
The criteria for selecting features is based on a QGIS expression. For more information about expressions see the Expressions.
See also
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[vector: any] |
Input vector layer |
Expression |
|
[expression] |
Expression to filter the input layer |
Modify current selection by |
|
[enumeration] Default: 0 |
How the selection of the algorithm should be managed. One of:
|
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[same as input] |
The input layer with features selected |
Python code
Algorithm ID: qgis:selectbyexpression
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.26.12. Select by location
Creates a selection in a vector layer.
The criteria for selecting features is based on the spatial relationship between each feature and the features in an additional layer.
Default menu:
See also
Exploring spatial relations
Geometric predicates are boolean functions used to determine the spatial relation a feature has with another by comparing whether and how their geometries share a portion of space.
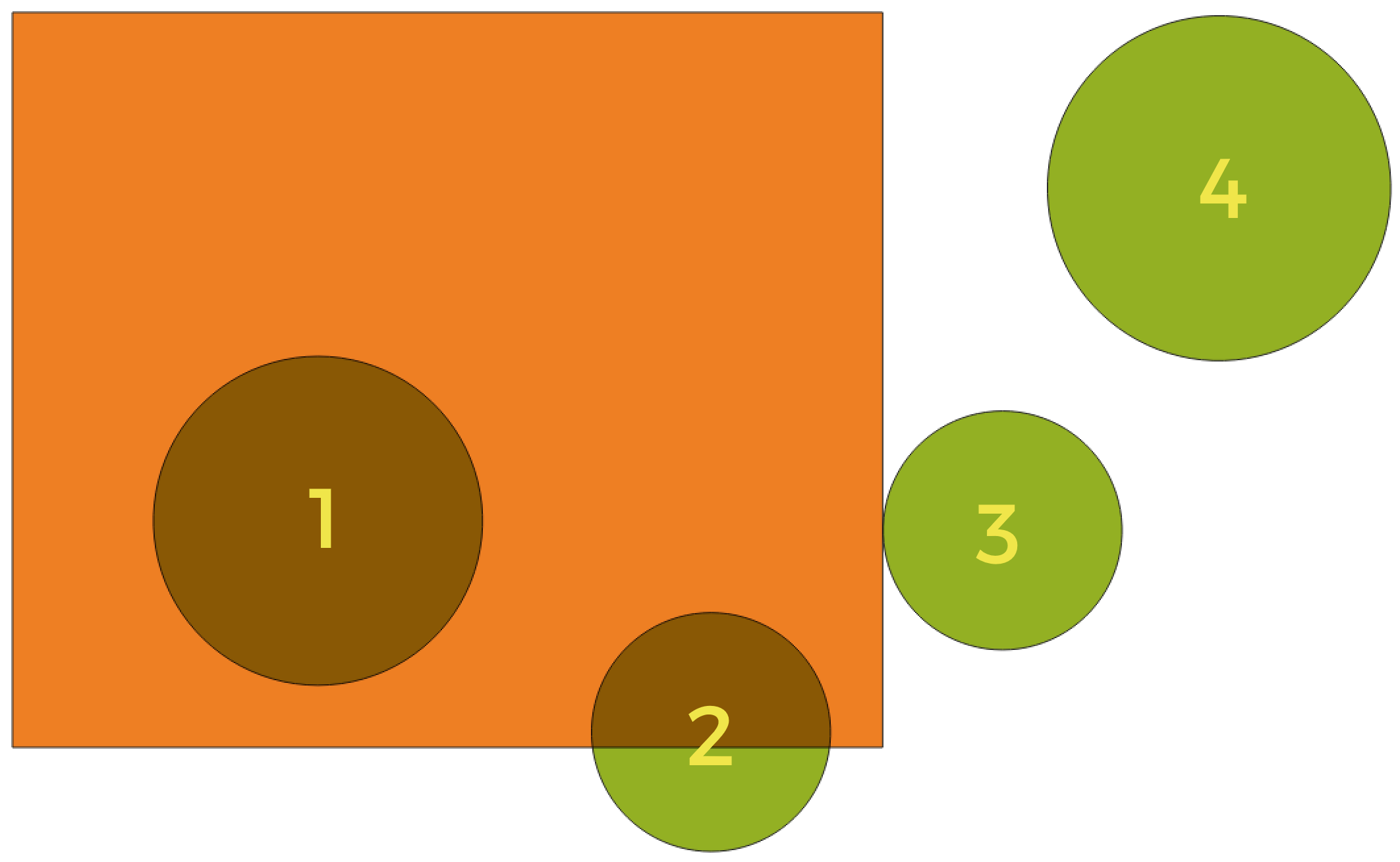
Fig. 24.134 Looking for spatial relations between layers
Using the figure above, we are looking for the green circles by spatially comparing them to the orange rectangle feature. Available geometric predicates are:
- Intersect
Tests whether a geometry intersects another. Returns 1 (true) if the geometries spatially intersect (share any portion of space - overlap or touch) and 0 if they don’t. In the picture above, this will return circles 1, 2 and 3.
- Contain
Returns 1 (true) if and only if no points of b lie in the exterior of a, and at least one point of the interior of b lies in the interior of a. In the picture, no circle is returned, but the rectangle would be if you would look for it the other way around, as it contains circle 1 completely. This is the opposite of are within.
- Disjoint
Returns 1 (true) if the geometries do not share any portion of space (no overlap, not touching). Only circle 4 is returned.
- Equal
Returns 1 (true) if and only if geometries are exactly the same. No circles will be returned.
- Touch
Tests whether a geometry touches another. Returns 1 (true) if the geometries have at least one point in common, but their interiors do not intersect. Only circle 3 is returned.
- Overlap
Tests whether a geometry overlaps another. Returns 1 (true) if the geometries share space, are of the same dimension, but are not completely contained by each other. Only circle 2 is returned.
- Are within
Tests whether a geometry is within another. Returns 1 (true) if geometry a is completely inside geometry b. Only circle 1 is returned.
- Cross
Returns 1 (true) if the supplied geometries have some, but not all, interior points in common and the actual crossing is of a lower dimension than the highest supplied geometry. For example, a line crossing a polygon will cross as a line (true). Two lines crossing will cross as a point (true). Two polygons cross as a polygon (false). In the picture, no circles will be returned.
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Select features from |
|
[vector: geometry] |
Input vector layer |
Where the features (geometric predicate) |
|
[enumeration] [list] Default: [0] |
Type of spatial relation the input feature should have with an intersect feature so that it could be selected. One or more of:
If more than one condition is chosen, at least one of them (OR operation) has to be met for a feature to be extracted. |
By comparing to the features from |
|
[vector: geometry] |
Intersection vector layer |
Modify current selection by |
|
[enumeration] Default: 0 |
How the selection of the algorithm should be managed. One of:
|
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[same as input] |
The input layer with features selected |
Python code
Algorithm ID: qgis:selectbylocation
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.26.13. Select within distance
creates a selection in a vector layer. Features are selected wherever they are within the specified maximum distance from the features in an additional reference layer.
See also
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Select features from |
|
[vector: geometry] |
Input vector layer to select features from |
By comparing to the features from |
|
[vector: geometry] |
Vector layer whose features closeness is used |
Where the features are within |
|
Default: 100.0 |
The maximum distance around reference features to select input features |
Modify current selection by |
|
[enumeration] Default: 0 |
How the selection of the algorithm should be managed. One of:
|
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[same as input] |
The input layer with features selected |
Python code
Algorithm ID: native:selectwithindistance
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.