23.1.1. Cartography
23.1.1.1. Combine style databases
Combines multiple QGIS style databases into a single style database. If items of the same type with the same name exist in different source databases these will be renamed to have unique names in the output combined database.
See also
23.1.1.1.1. Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input databases |
|
[file] [list] |
Files containing QGIS style items |
Objects to combine |
|
[enumeration] [list] |
Types of style items in the input databases you would like to put in the new database. These can be:
|
Output style database |
|
[file] Default: |
Output
The file encoding can also be changed here. |
23.1.1.1.2. Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Color ramp count |
|
[number] |
|
Label settings count |
|
[number] |
|
Output style database |
|
[file] |
Output |
Symbol count |
|
[number] |
|
Text format count |
|
[number] |
23.1.1.1.3. Python code
Algorithm ID: qgis:combinestyles
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
23.1.1.2. Create categorized renderer from styles
Sets a vector layer’s renderer to a categorized renderer using matching symbols from a style database. If no style file is specified, symbols from the user’s current symbol library are used instead.
A specified expression or field is used to create categories for the renderer. Each category is individually matched to the symbols which exist within the specified QGIS XML style database. Whenever a matching symbol name is found, the category’s symbol will be set to this matched symbol.
If desired, outputs can also be tables containing lists of the categories which could not be matched to symbols, and symbols which were not matched to categories.
23.1.1.2.1. Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[vector: any] |
Vector layer to apply a categorized style to |
Categorize using expression |
|
[expression] |
Field or expression to categorize the features |
Style database (leave blank to use saved symbols) |
|
[file] |
File ( |
Use case-sensitive match to symbol names |
|
[boolean] Default: False |
If True (checked), applies a case sensitive comparison between the categories and symbols names |
Ignore non-alphanumeric characters while matching |
|
[boolean] Default: False |
If True (checked), non-alphanumeric characters in the categories and symbols names will be ignored, allowing greater tolerance during the match. |
Non-matching categories Optional |
|
[table] Default: |
Output table for categories which do not match any symbol in the database. One of:
The file encoding can also be changed here. |
Non-matching symbol names Optional |
|
[table] Default: |
Output table for symbols from the provided style database which do not match any category. One of:
The file encoding can also be changed here. |
23.1.1.2.2. Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Non-matching categories |
|
[table] |
Lists categories which could not be matched to any symbol in the provided style database |
Non-matching symbol names |
|
[table] |
Lists symbols from the provided style database which could not match any category |
Categorized layer |
|
[same as input] |
The input vector layer with the categorized style applied. No new layer is output. |
23.1.1.2.3. Python code
Algorithm ID: qgis:categorizeusingstyle
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
23.1.1.3. Create style database from project
Extracts all style objects (symbols, color ramps, text formats and label settings) from a QGIS project.
The extracted symbols are saved to a QGIS style database (XML
format),
which can be managed and imported via the Style Manager
dialog.
See also
23.1.1.3.1. Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input project (leave blank to use current) Optional |
|
[file] |
A QGIS project file to extract the style items from |
Objects to extract |
|
[enumeration] [list] |
Types of style items in the input project you would like to put in the new database. These can be:
|
Output style database |
|
[file] Default: |
Specify the output
The file encoding can also be changed here. |
23.1.1.3.2. Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Color ramp count |
|
[number] |
Number of color ramps |
Label settings count |
|
[number] |
Number of label settings |
Output style database |
|
[file] |
Output |
Symbol count |
|
[number] |
Number of symbols |
Text format count |
|
[number] |
Number of text formats |
23.1.1.3.3. Python code
Algorithm ID: qgis:stylefromproject
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
23.1.1.4. Print layout map extent to layer
Creates a polygon layer containing the extent of a print layout map item (or items), with attributes specifying the map size (in layout units, i.e. the reference map units), scale and rotation.
If the map item parameter is specified, then only the matching map extent will be exported. If it is not specified, all map extents from the layout will be exported.
Optionally, a specific output CRS can be specified. If it is not specified, the original map item CRS will be used.
Label |
Name |
Type |
Description |
---|---|---|---|
Print layout |
|
[enumeration] |
A print layout in the current project |
Map item Optional |
|
[enumeration] Default: All the map items |
The map item(s) whose information you want to extract. If none is provided then all the map items are processed. |
Overrride CRS Optional |
|
[crs] Default: The layout CRS |
Select the CRS for the layer in which the information will be reported. |
Extent |
|
[vector: polygon] Default: |
Specify the output vector layer for the extent(s). One of:
The file encoding can also be changed here. |
23.1.1.4.1. Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Map height |
|
[number] |
|
Extent |
|
[vector: polygon] |
Output polygon vector layer containing extents of all the input layout map item(s) |
Map rotation |
|
[number] |
|
Map scale |
|
[number] |
|
Map width |
|
[number] |
23.1.1.4.2. Python code
Algorithm ID: qgis:printlayoutmapextenttolayer
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
23.1.1.5. Topological coloring
Assigns a color index to polygon features in such a way that no adjacent polygons share the same color index, whilst minimizing the number of colors required.
The algorithm allows choice of method to use when assigning colors.
A minimum number of colors can be specified if desired. The color index is saved to a new attribute named color_id.
The following example shows the algorithm with four different colors chosen; as you can see each color class has the same amount of features.
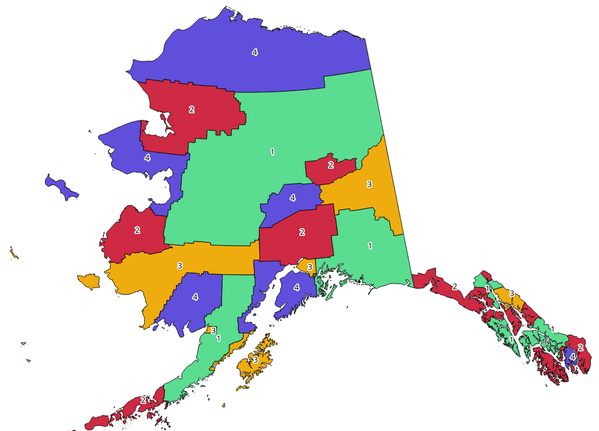
Fig. 23.1 Topological colors example
23.1.1.5.1. Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[vector: polygon] |
The input polygon layer |
Minimum number of colors |
|
[number] Default: 4 |
The minimum number of colors to assign. Minimum 1, maximum 1000. |
Minimum distance between features |
|
[number] Default: 0.0 |
Prevent nearby (but non-touching) features from being assigned equal colors. Minimum 0.0. |
Balance color assignment |
|
[enumeration] Default: 0 |
Options are:
|
Colored |
|
[vector: polygon] Default: |
Specify the output layer. One of:
The file encoding can also be changed here. |
23.1.1.5.2. Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Colored |
|
[vector: polygon] |
Polygon vector layer with an added |
23.1.1.5.3. Python code
Algorithm ID: qgis:topologicalcoloring
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.