14. Authentication infrastructure
Hint
The code snippets on this page need the following imports if you’re outside the pyqgis console:
1from qgis.core import (
2 QgsApplication,
3 QgsRasterLayer,
4 QgsAuthMethodConfig,
5 QgsDataSourceUri,
6 QgsPkiBundle,
7 QgsMessageLog,
8)
9
10from qgis.gui import (
11 QgsAuthAuthoritiesEditor,
12 QgsAuthConfigEditor,
13 QgsAuthConfigSelect,
14 QgsAuthSettingsWidget,
15)
16
17from qgis.PyQt.QtWidgets import (
18 QWidget,
19 QTabWidget,
20)
21
22from qgis.PyQt.QtNetwork import QSslCertificate
14.1. Introduction
User reference of the Authentication infrastructure can be read in the User Manual in the Authentication System Overview paragraph.
This chapter describes the best practices to use the Authentication system from a developer perspective.
The authentication system is widely used in QGIS Desktop by data providers whenever credentials are required to access a particular resource, for example when a layer establishes a connection to a Postgres database.
There are also a few widgets in the QGIS gui library that plugin developers can use to easily integrate the authentication infrastructure into their code:
A good code reference can be read from the authentication infrastructure tests code.
Warning
Due to the security constraints that were taken into account during the authentication infrastructure design, only a selected subset of the internal methods are exposed to Python.
14.2. Glossary
Here are some definition of the most common objects treated in this chapter.
- Master Password
Password to allow access and decrypt credential stored in the QGIS Authentication DB
- Authentication Database
A Master Password crypted sqlite db
qgis-auth.db
where Authentication Configuration are stored. e.g user/password, personal certificates and keys, Certificate Authorities- Authentication DB
- Authentication Configuration
A set of authentication data depending on Authentication Method. e.g Basic authentication method stores the couple of user/password.
- Authentication Config
- Authentication Method
A specific method used to get authenticated. Each method has its own protocol used to gain the authenticated level. Each method is implemented as shared library loaded dynamically during QGIS authentication infrastructure init.
14.3. QgsAuthManager the entry point
The QgsAuthManager
singleton
is the entry point to use the credentials stored in the QGIS encrypted
Authentication DB, i.e. the qgis-auth.db
file under the
active user profile folder.
This class takes care of the user interaction: by asking to set a master password or by transparently using it to access encrypted stored information.
14.3.1. Init the manager and set the master password
The following snippet gives an example to set master password to open the access to the authentication settings. Code comments are important to understand the snippet.
1authMgr = QgsApplication.authManager()
2
3# check if QgsAuthManager has already been initialized... a side effect
4# of the QgsAuthManager.init() is that AuthDbPath is set.
5# QgsAuthManager.init() is executed during QGIS application init and hence
6# you do not normally need to call it directly.
7if authMgr.authenticationDatabasePath():
8 # already initialized => we are inside a QGIS app.
9 if authMgr.masterPasswordIsSet():
10 msg = 'Authentication master password not recognized'
11 assert authMgr.masterPasswordSame("your master password"), msg
12 else:
13 msg = 'Master password could not be set'
14 # The verify parameter checks if the hash of the password was
15 # already saved in the authentication db
16 assert authMgr.setMasterPassword("your master password",
17 verify=True), msg
18else:
19 # outside qgis, e.g. in a testing environment => setup env var before
20 # db init
21 os.environ['QGIS_AUTH_DB_DIR_PATH'] = "/path/where/located/qgis-auth.db"
22 msg = 'Master password could not be set'
23 assert authMgr.setMasterPassword("your master password", True), msg
24 authMgr.init("/path/where/located/qgis-auth.db")
14.3.2. Populate authdb with a new Authentication Configuration entry
Any stored credential is a Authentication Configuration instance of the
QgsAuthMethodConfig
class accessed using a unique string like the following one:
authcfg = 'fm1s770'
that string is generated automatically when creating an entry using the QGIS API or GUI, but it might be useful to manually set it to a known value in case the configuration must be shared (with different credentials) between multiple users within an organization.
QgsAuthMethodConfig
is the base class
for any Authentication Method.
Any Authentication Method sets a configuration hash map where authentication
information will be stored. Hereafter a useful snippet to store PKI-path
credentials for a hypothetical alice user:
1authMgr = QgsApplication.authManager()
2# set alice PKI data
3config = QgsAuthMethodConfig()
4config.setName("alice")
5config.setMethod("PKI-Paths")
6config.setUri("https://example.com")
7config.setConfig("certpath", "path/to/alice-cert.pem" )
8config.setConfig("keypath", "path/to/alice-key.pem" )
9# check if method parameters are correctly set
10assert config.isValid()
11
12# register alice data in authdb returning the ``authcfg`` of the stored
13# configuration
14authMgr.storeAuthenticationConfig(config)
15newAuthCfgId = config.id()
16assert newAuthCfgId
14.3.2.1. Available Authentication methods
Authentication Method libraries are loaded dynamically during authentication manager init. Available authentication methods are:
Basic
User and password authenticationEsriToken
ESRI token based authenticationIdentity-Cert
Identity certificate authenticationOAuth2
OAuth2 authenticationPKI-Paths
PKI paths authenticationPKI-PKCS#12
PKI PKCS#12 authentication
14.3.2.3. Manage PKI bundles with QgsPkiBundle
A convenience class to pack PKI bundles composed on SslCert, SslKey and CA
chain is the QgsPkiBundle
class. Hereafter a snippet to get password protected:
1# add alice cert in case of key with pwd
2caBundlesList = [] # List of CA bundles
3bundle = QgsPkiBundle.fromPemPaths( "/path/to/alice-cert.pem",
4 "/path/to/alice-key_w-pass.pem",
5 "unlock_pwd",
6 caBundlesList )
7assert bundle is not None
8# You can check bundle validity by calling:
9# bundle.isValid()
Refer to QgsPkiBundle
class documentation
to extract cert/key/CAs from the bundle.
14.3.3. Remove an entry from authdb
We can remove an entry from Authentication Database using it’s
authcfg
identifier with the following snippet:
authMgr = QgsApplication.authManager()
authMgr.removeAuthenticationConfig( "authCfg_Id_to_remove" )
14.3.4. Leave authcfg expansion to QgsAuthManager
The best way to use an Authentication Config stored in the
Authentication DB is referring it with the unique identifier
authcfg
. Expanding, means convert it from an identifier to a complete
set of credentials.
The best practice to use stored Authentication Configs, is to leave it
managed automatically by the Authentication manager.
The common use of a stored configuration is to connect to an authentication
enabled service like a WMS or WFS or to a DB connection.
Note
Take into account that not all QGIS data providers are integrated with the
Authentication infrastructure. Each authentication method, derived from the
base class QgsAuthMethod
and support a different set of Providers. For example the certIdentity()
method supports the following list
of providers:
authM = QgsApplication.authManager()
print(authM.authMethod("Identity-Cert").supportedDataProviders())
Sample output:
['ows', 'wfs', 'wcs', 'wms', 'postgres']
For example, to access a WMS service using stored credentials identified with
authcfg = 'fm1s770'
, we just have to use the authcfg
in the data source
URL like in the following snippet:
1authCfg = 'fm1s770'
2quri = QgsDataSourceUri()
3quri.setParam("layers", 'usa:states')
4quri.setParam("styles", '')
5quri.setParam("format", 'image/png')
6quri.setParam("crs", 'EPSG:4326')
7quri.setParam("dpiMode", '7')
8quri.setParam("featureCount", '10')
9quri.setParam("authcfg", authCfg) # <---- here my authCfg url parameter
10quri.setParam("contextualWMSLegend", '0')
11quri.setParam("url", 'https://my_auth_enabled_server_ip/wms')
12rlayer = QgsRasterLayer(str(quri.encodedUri(), "utf-8"), 'states', 'wms')
In the upper case, the wms
provider will take care to expand authcfg
URI parameter with credential just before setting the HTTP connection.
Warning
The developer would have to leave authcfg
expansion to the QgsAuthManager
, in this way he will be sure that expansion is not done too early.
Usually an URI string, built using the QgsDataSourceURI
class, is used to set a data source in the following way:
authCfg = 'fm1s770'
quri = QgsDataSourceUri("my WMS uri here")
quri.setParam("authcfg", authCfg)
rlayer = QgsRasterLayer( quri.uri(False), 'states', 'wms')
Note
The False
parameter is important to avoid URI complete expansion of the
authcfg
id present in the URI.
14.3.4.1. PKI examples with other data providers
Other example can be read directly in the QGIS tests upstream as in test_authmanager_pki_ows or test_authmanager_pki_postgres.
14.4. Adapt plugins to use Authentication infrastructure
Many third party plugins are using httplib2 or other Python networking libraries to manage HTTP
connections instead of integrating with QgsNetworkAccessManager
and its related Authentication Infrastructure integration.
To facilitate this integration a helper Python function has been created
called NetworkAccessManager
. Its code can be found here.
This helper class can be used as in the following snippet:
1http = NetworkAccessManager(authid="my_authCfg", exception_class=My_FailedRequestError)
2try:
3 response, content = http.request( "my_rest_url" )
4except My_FailedRequestError, e:
5 # Handle exception
6 pass
14.5. Authentication GUIs
In this paragraph are listed the available GUIs useful to integrate authentication infrastructure in custom interfaces.
14.5.1. GUI to select credentials
If it’s necessary to select a Authentication Configuration from the set
stored in the Authentication DB it is available in the GUI class
QgsAuthConfigSelect
.
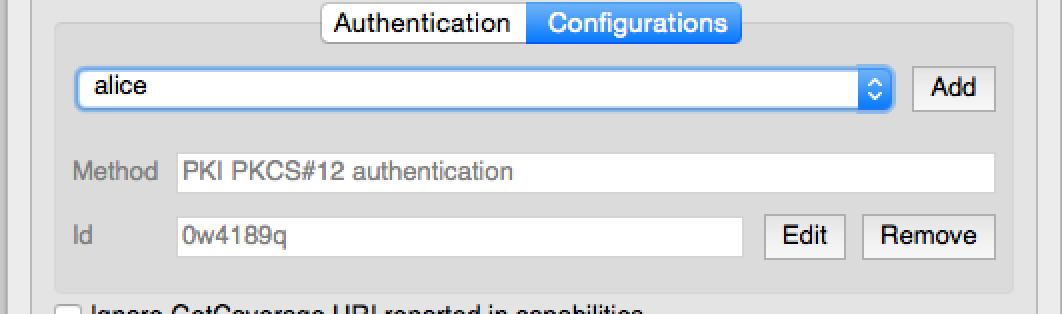
and can be used as in the following snippet:
1# create the instance of the QgsAuthConfigSelect GUI hierarchically linked to
2# the widget referred with `parent`
3parent = QWidget() # Your GUI parent widget
4gui = QgsAuthConfigSelect( parent, "postgres" )
5# add the above created gui in a new tab of the interface where the
6# GUI has to be integrated
7tabGui = QTabWidget()
8tabGui.insertTab( 1, gui, "Configurations" )
The above example is taken from the QGIS source code. The second parameter of the GUI constructor refers to data provider type. The parameter is used to restrict the compatible Authentication Methods with the specified provider.
14.5.2. Authentication Editor GUI
The complete GUI used to manage credentials, authorities and to access to
Authentication utilities is managed by the
QgsAuthEditorWidgets
class.
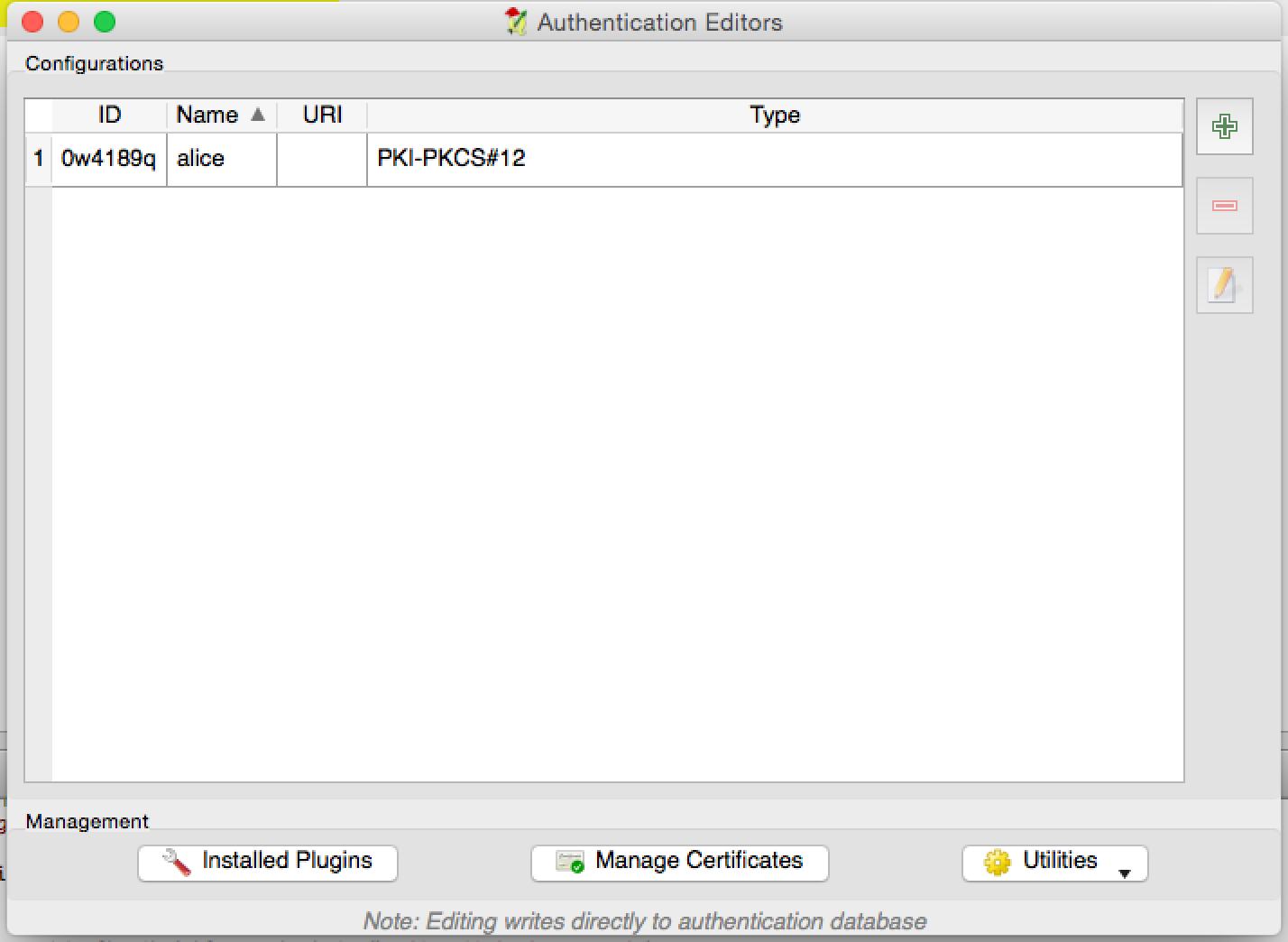
and can be used as in the following snippet:
1# create the instance of the QgsAuthEditorWidgets GUI hierarchically linked to
2# the widget referred with `parent`
3parent = QWidget() # Your GUI parent widget
4gui = QgsAuthConfigSelect( parent )
5gui.show()
An integrated example can be found in the related test.