24.1.13. Point Cloud Conversion
Note
These algorithms are only available if QGIS uses the PDAL library version 2.5.0 or newer.
24.1.13.1. Convert format
Converts a point cloud to a different file format, e.g. creates a compressed .LAZ
.
Parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[point cloud] |
Input point cloud layer to convert |
Converted |
|
[point cloud] Default: |
Specify the point cloud file to use as output. One of:
|
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Converted |
|
[point cloud] |
Output point cloud layer in a modified file format.
Currently supported formats are |
Python code
Algorithm ID: pdal:convertformat
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.13.2. Export to raster
Exports point cloud data to a 2D raster grid having cell size of given resolution, writing values from the specified attribute.
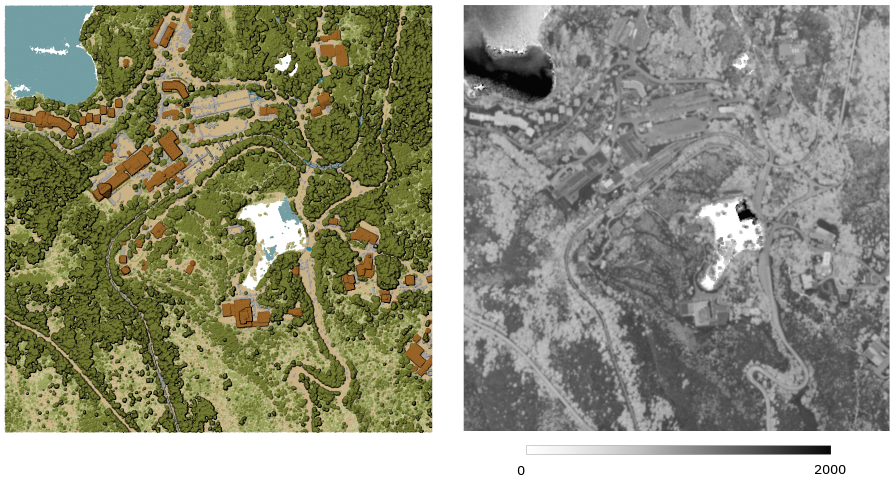
Fig. 24.10 Raster output using Intensity attribute of points
Parameters
Basic parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[point cloud] |
Input point cloud layer to export |
Attribute |
|
[field] [enumeration] |
A Field of the point cloud layer to extract the values from |
Resolution of the density raster |
|
[numeric: double] Default: 1.0 |
Cell size of the output raster |
Tile size for parallel runs |
|
[numeric: integer] Default: 1000 |
|
Exported |
|
[raster] Default: |
Specify the raster file to export the data to. One of:
|
Advanced parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Filter expression Optional |
|
[expression] |
A PDAL expression for selecting a subset of features in the point cloud data |
Cropping extent Optional |
|
[extent] |
A map extent for selecting a subset of features in the point cloud data Available methods are:
|
X origin of a tile for parallel runs Optional |
|
[numeric: double] |
|
Y origin of a tile for parallel runs Optional |
|
[numeric: double] |
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Exported |
|
[raster] |
Output raster layer features of the point cloud layer are exported to.
Currently supported format is |
Python code
Algorithm ID: pdal:exportraster
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.13.3. Export raster (using triangulation)
Exports point cloud data to a 2D raster grid using a triangulation of points and then interpolating cell values from triangles.
Note
Using this algorithm can be slower if you are dealing with a large dataset. If your point cloud is dense, you can export your ground points as a raster using the Export to raster algorithm.
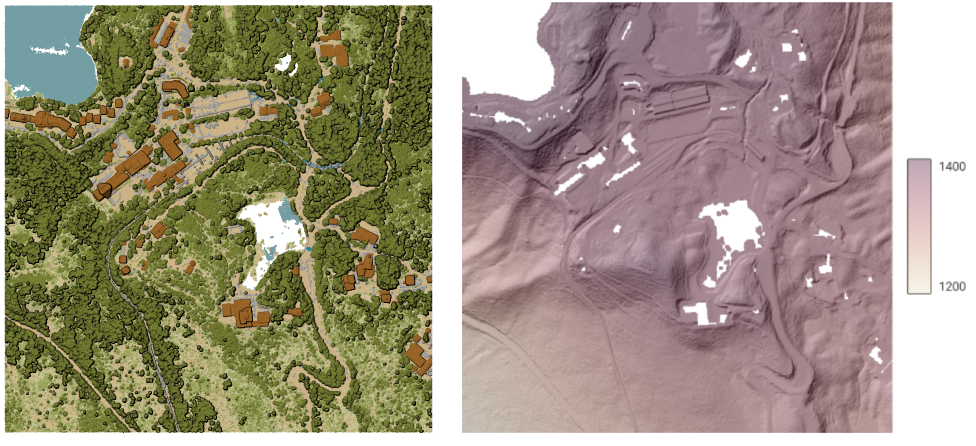
Fig. 24.11 Terrain raster output generated by point cloud triangulation
Parameters
Basic parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[point cloud] |
Input point cloud layer to export |
Resolution of the density raster |
|
[numeric: double] Default: 1.0 |
Cell size of the output raster |
Tile size for parallel runs |
|
[numeric: integer] Default: 1000 |
|
Exported |
|
[raster] Default: |
Specify the raster file to export the data to. One of:
|
Advanced parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Filter expression Optional |
|
[expression] |
A PDAL expression for selecting a subset of features in the point cloud data |
Cropping extent Optional |
|
[extent] |
A map extent for selecting a subset of features in the point cloud data Available methods are:
|
X origin of a tile for parallel runs Optional |
|
[numeric: double] |
|
Y origin of a tile for parallel runs Optional |
|
[numeric: double] |
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Exported (using triangulation) |
|
[raster] |
Output raster layer features of the point cloud layer are exported to.
Currently supported format is |
Python code
Algorithm ID: pdal:exportrastertin
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.
24.1.13.4. Export to vector
Exports point cloud data to a vector layer with 3D points (a GeoPackage), optionally with extra attributes.
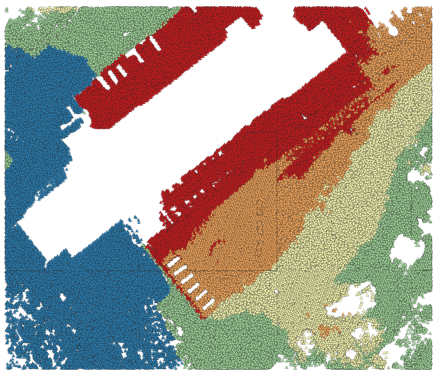
Fig. 24.12 Exporting point cloud (ground points) to a vector layer styled based on the elevation
Parameters
Basic parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Input layer |
|
[point cloud] |
Input point cloud layer to export |
Attribute Optional |
|
[field] [list] |
One or more fields of the point cloud layer to export with the points. |
Exported |
|
[vector] Default: |
Specify the vector file to export the data to. One of:
|
Advanced parameters
Label |
Name |
Type |
Description |
---|---|---|---|
Filter expression Optional |
|
[expression] |
A PDAL expression for selecting a subset of features in the point cloud data |
Cropping extent Optional |
|
[extent] |
A map extent for selecting a subset of features in the point cloud data Available methods are:
|
Outputs
Label |
Name |
Type |
Description |
---|---|---|---|
Exported |
|
[vector] |
Output vector layer features of the point cloud layer are exported to.
Currently supported format is |
Python code
Algorithm ID: pdal:exportvector
import processing
processing.run("algorithm_id", {parameter_dictionary})
The algorithm id is displayed when you hover over the algorithm in the Processing Toolbox. The parameter dictionary provides the parameter NAMEs and values. See Using processing algorithms from the console for details on how to run processing algorithms from the Python console.